Proposal for consolidated permissions
Problem:
More and more really neat features are coming to web applications. These features include local file system access, access to cameras and microphones, geo locations services and many more. To minimize security risks and to protect the user’s private data current web browser generations ask for permission before they grant access to these services. This gets problematic when a browser has to ask for several permissions at the same time. Figure 1 illustrates this behaviour as seen in Google Chrome. As shown in Figure 2 Mozilla Firefox handles this even worse as the two dialogs overlap.
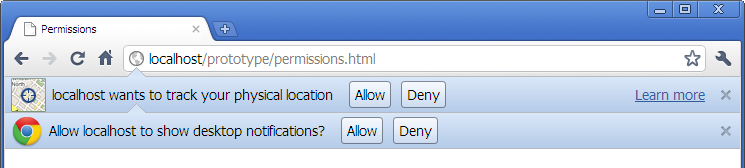

In both cases the permission dialogs might confuse the user and the more permissions have to be asked for the more confusing this might get. It would therefore be very nice to have a consolidated permission dialog asking for all permissions at once or at least multiple permissions at the same time.
Normally, this would not concern any web standard since this would be strictly an UI issue, but the problem is not that easy to solve at UI level because permissions are not questioned while the application is loading but while each individual feature is used for the first time.
Proposed solution:
I recently installed an Android application on a mobile phone and saw that it shows the user which features it would like to access. The user sees this all at once and can transparently view the requirements for this application. Therefore, I suggest a similar behaviour for web applications.
I came across the article “Device API permission management“ by dough which allows an applications to ask for multiple permissions at once. I like this idea because it would allow asking for related permissions or permissions that are required for the same application feature simultaniously. This way an application could also ask for all the permissions it ever needs on start-up. Listing 1 is taken from dough's article and shows how to request multiple permissions.
navigator.permissions.requestPermissions("notifications, offline-storage", callback); function callback(result) { if (result["notifications"].granted) // great, we can show notifications. }
An application could even check back for permissions to find out if they where granted by the user.
navigator.permissions.checkPermissions("notifications, offline-storage", callback);
To illustrate the benefit of this I created two mock-up screens that show how such permission requests could be presented to the user. Figure 3 shows what happens if a web application runs the code from listing 1 and requests two permissions. The permission dialog could pop-up listing only the newly requested permissions. A "Allow all" button could make this quick and convenient for the user.

Once users click on the arrow placed on bottom of the dialog they would have access to the full list of permissions this web applications requests or has requested. Figure 4 shows how this could look like. The yellow highlighted entries are the once that where also visible in the "smaller" version of the dialog. They are highligthed because those are the most recently requested once.

Another thing that came up while discussing this is that the user might not know why to grant a permission. To solve this the above javascript listing 1 could be extended to also hold a small custom-definable description why the application needs which permission. Listing 3 shows the code which could be used to ask for two permissions at once, each provided with a short reason.
navigator.permissions.requestPermissions( { permission: "notifications", reason: "Notify the user of incoming offers while the browser window is minimized" }, { permission: "offline-storage", reason: "Save the last three orders to view when offline" }, callback ); function callback(result) { if (result["notifications"].granted) // great, we can show notifications. }